들어가며
styled components를 이용하여 제작한 노트앱 프로젝트를 배포하고 모니터링하던 중에 이상한 오류를 발견했다.
pc환경에선 최신순, 오래된순 정렬이 정상적으로 작동하는데 모바일(아이폰 mini12 기종)로 확인해보니 정렬이 안되는
오류가 있었다.
먼저 어떤 형식으로 날짜를 설정했는지 보고 오류를 찾아보도록 하겠다.
toLocaleDateString()
// POST:CREATE
if(!contRef.current.value){
if(window.confirm("내용이 없습니다. 그대로 저장하시겠습니까?")){
axios.post(`${process.env.REACT_APP_API_URL}/notes`,{
(...)
day:new Date().toLocaleDateString(),
timestamp:new Date().getTime()
(...)
}
해당 메서드를 사용하면 new Date()의 기준으로 2024. 7. 14 같은 형식의 날짜가 표시된다.
또 날짜가 같은 콘텐츠에서도 정렬을 하기 위해 초단위(timestamp)도 함께 POST 해준다.
sort 정렬
useEffect(()=>{
const result = data.sort((a,b)=>{
if(a.day === b.day){
return b.timestamp - a.timestamp;
}
return new Date(b.day) - new Date(a.day)}
)
setItem(result);
},[data]);
처음 App.js 컴포넌트를 렌더링할 때 정렬 상태를 useEffect 훅을 사용하여 세팅해준다.
sort 정렬은 b - a로 호출하면 내림차순(최신순), a - b로 호출하면 오름차순(오래된순)으로 정렬한다.
만약 날짜가 같다면 그 안에서도 timestamp로 정렬하는 조건문을 추가해준다.
select 태그로 정렬 상태 변경
# Home.js
export default function Home({ latestData }){
function handleOnChangeSelect(e){
latestData(e.target.value)
}
(...)
<select onChange={handleOnChangeSelect}>
<option value={'latest'}>최신순</option>
<option value={'oldest'}>오래된순</option>
</select>
# App.js
function latestData(e){
setLatest(e); //props로 받아온 select value
if(e === 'latest'){
const result = item.sort((a,b)=>{
if(a.day === b.day){
return b.timestamp - a.timestamp;
}
return new Date(b.day) - new Date(a.day);
})
setItem(result); //데이터 state 상태변경
}else{
const result = item.sort((a,b)=>{
if(a.day === b.day){
return a.timestamp - b.timestamp;
}
return new Date(a.day) - new Date(b.day);
})
setItem(result); //데이터 state 상태변경
}
}
만약 select 태그를 사용하여 정렬 상태를 변경하고 싶다면 위와 같이 조건문을 추가해준다.
이런식으로 세팅하면 2024. 1. 12의 형식으로 정상적으로 정렬이 된다.
날짜 형식 꾸미기
여기서 2024.01.12 처럼 월, 일이 한자리수면 앞에 0이 추가되도록 함수를 추가했다.
# /src/components/util.js
export const getFormattedDate = (targetDate)=>{
let year = targetDate.getFullYear();
let month = targetDate.getMonth() +1;
let date = targetDate.getDate();
if(month < 10){
month = `0${month}`;
}
if(date<10){
date = `0${date}`;
}
return `${year}.${month}.${date}`
}
# /src/pages/New.js
import { getFormattedDate } from "../components/util"; //함수 불러오기
if(!contRef.current.value){
if(window.confirm("내용이 없습니다. 그대로 저장하시겠습니까?")){
axios.post(`${process.env.REACT_APP_API_URL}/notes`,{
(...)
day:getFormattedDate(new Date()), //오늘 날짜로 적용
timestamp:new Date().getTime()
(...)
}
return `${year}.${month}.${date}`으로 년 월 일 사이에 점을 배치하여 2024.01.17로 정렬했다.
아이폰(IOS)환경 날짜 정렬 오류
그 러 나
안드로이드, PC 모두 동일하게 잘 작동되지만 아이폰(IOS) 환경에선 정렬이 전혀 작동되지 않는 오류가 있었다.
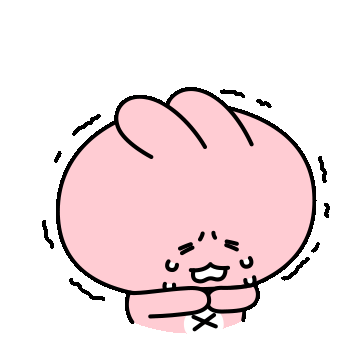
아이폰을 안썼다면 몰랐을 오류였다.
찾아보니 아이폰은 날짜를 ' . '(점)으로 구분하면 정렬이 안된다고 한다.
2024-01-10 또는 2024/01/10 형식으로 구분해야했다.
위의 getFormattedDate 함수에서 return 부분의 ' . '을 ' / '로 교체했다.
export const getFormattedDate = (targetDate)=>{
(...)
return `${year}/${month}/${date}`
}
보통은 ' - '를 사용한다고 하는데 나는 점으로 구분하고 싶었기에
정렬할 날짜는 ' / '를 적용하고 보이는 날짜는 ' . '으로 보이도록 수정했다.
최종 수정
# /src/conponents/util.js //내용추가
export const getFormattedDate = (targetDate)=>{ //정렬 버전 날짜
(...)
return `${year}/${month}/${date}`
}
export const getShowDate = (targetDate)=>{ //보이는 날짜
let year = targetDate.getFullYear();
let month = targetDate.getMonth() +1;
let date = targetDate.getDate();
if(month < 10){
month = `0${month}`;
}
if(date<10){
date = `0${date}`;
}
return `${year}.${month}.${date}`
}
# /src/pages/New.js //POST
(...)
axios.post(`${process.env.REACT_APP_API_URL}/notes`,{
(...)
day:getFormattedDate(new Date()),
showDay:getShowDate(new Date()),
timestamp:new Date().getTime()
(...)
}
# /src/components/NoteItem.js //보이는 날짜 props 수정
<Link to={`/view/${it.id}`} >
<h1>{it.title}</h1>
<p>{it.showDay}</p> // it.day -> it.showDay 교체
</Link>
'Study > React' 카테고리의 다른 글
[React] Next.js 프로젝트 초기 세팅 (0) | 2024.07.26 |
---|---|
[React] Next.js 란? (Next.js와 React.js의 차이점) (1) | 2024.07.24 |
[React] .env로 경로 숨기기 (배포, 개발) (1) | 2024.07.18 |
[React] css 말줄임 처리 & 리액트 툴팁 스타일링하기 (React Tooltip) (0) | 2024.07.16 |
[React] styled-components로 다크모드 만들기 (placeholder, after, before...) (0) | 2024.07.12 |